概要
コンソールアプリから「天気予報API」を叩き、東京の天気予報を取得します。
環境
- Windows10
- .Net6
インストール
Web API クライアントライブラリ
パッケージマネージャーコンソールで次のコマンドを実行しインストールを行います。
Install-Package Microsoft.AspNet.WebApi.Client
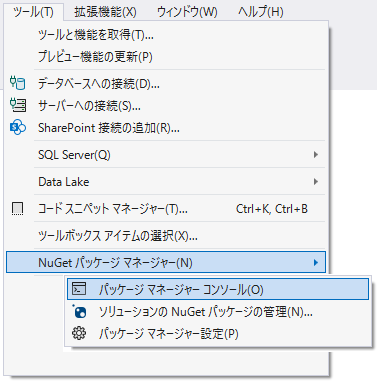
呼び出し
リソース取得用のモデルクラスを作成
入れ子になっているJSONにも対応できるように、複数のクラスを用います。
public class Weather
{
public string publicTimeFormatted { get; set; }
public Description description { get; set; }
public List<Forecasts> forecasts { get; set; }
}
public class Description
{
public string publicTimeFormatted { get; set; }
public string bodyText { get; set; }
}
public class Forecasts
{
public string date { get; set; }
public string dateLabel { get; set; }
public string telop { get; set; }
public Detail detail { get; set; }
}
public class Detail
{
public string weather { get; set; }
public string wind { get; set; }
public string wave { get; set; }
}
HttpClientの作成
HttpClient は 毎回新しいソケットを開かなくて良いように、インスタンスを static 変数で持っておき、オブジェクトを使いまわすよう実装しています。
詳細は「公式ドキュメント」を参照してください。
static HttpClient client = new HttpClient();
static async Task RunAsync()
{
//HTTP 要求のベース URI を設定します。
client.BaseAddress = new Uri(baseURL);
client.DefaultRequestHeaders.Accept.Clear();
//JSON形式でデータを送信する
client.DefaultRequestHeaders.Accept.Add(
new MediaTypeWithQualityHeaderValue("application/json"));
try
{
var tokyoCd = 130010;
// GetAsync メソッドは HTTP GET 要求を送信します。
var weather = await GetWeatherAsync(baseURL + $"/city/{tokyoCd}");
// 取得結果の表示
Console.WriteLine(weather.title);
Console.WriteLine($"明日({weather.forecasts[1].date})の天気は{weather.forecasts[1].telop}です。");
Console.WriteLine($"風速 {weather.forecasts[1].detail.wave}");
Console.WriteLine($"{weather.forecasts[1].detail.wind}になるでしょう。");
Console.WriteLine($"予報発生時刻: {weather.publicTimeFormatted}");
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
Console.ReadLine();
}
GET要求を送信し、リソースを取得
GetAsync メソッドが完了した場合、HTTP 応答を含む HttpResponseMessage が返却され、成功コードの場合、天気予報の情報がJSONとして格納されます。
ReadAsAsyncを呼び出し、デシリアライズを行います。
static async Task<Weather> GetWeatherAsync(string path)
{
Weather weather = null;
HttpResponseMessage response = await client.GetAsync(path);
if (response.IsSuccessStatusCode)
{
weather = await response.Content.ReadAsAsync<Weather>();
}
return weather;
}
// MAIN
static void Main(string[] args)
{
RunAsync().GetAwaiter().GetResult();
}